Ch1ΒΆ
[1]:
import importlib
from distutils.version import LooseVersion
# check that all packages are installed (see requirements.txt file)
required_packages = {'jupyter',
'numpy',
'matplotlib',
'ipywidgets',
'scipy',
'pandas',
'numba',
'multiprocess',
'SimpleITK'
}
problem_packages = list()
# Iterate over the required packages: If the package is not installed
# ignore the exception.
for package in required_packages:
try:
p = importlib.import_module(package)
except ImportError:
problem_packages.append(package)
if len(problem_packages) == 0:
print('All is well.')
else:
print('The following packages are required but not installed: ' \
+ ', '.join(problem_packages))
All is well.
[2]:
import matplotlib.pyplot as plt
import SimpleITK as sitk
[3]:
image = sitk.Image(256, 128, 64, sitk.sitkInt16)
image_2D = sitk.Image(64, 64, sitk.sitkFloat32)
image_2D = sitk.Image([32,32], sitk.sitkUInt32)
image_RGB = sitk.Image([128,128], sitk.sitkVectorUInt8, 3)
nda = sitk.GetArrayFromImage(image)
plt.imshow(nda[:,:,0])
[3]:
<matplotlib.image.AxesImage at 0x7f6042bb3400>
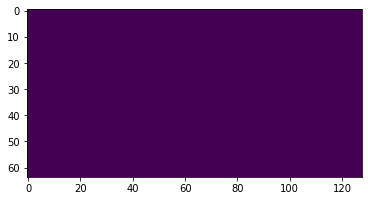